Plot Elements
Prerequisites
Concepts |
Importance |
Notes |
---|---|---|
Necessary |
||
Necessary |
Time to learn: 10 minutes
import xarray as xr
import numpy as np
import matplotlib.pyplot as plt
import geocat.viz as gv
Data
The first piece of data visualization is the data!
Let’s generate some dummy data to work with:
x = np.linspace(0, 20, 1000)
y1 = np.sin(x)
y2 = np.cos(x)
Figure
The figure is the object that contains your entire visualization. Creating a figure tends to be the first step in plotting, even if it doesn’t currently show anything:
fig = plt.figure(figsize=(9.5, 8))
<Figure size 950x800 with 0 Axes>
Axis
We then add axes to our plot. You can add multiple axes to one plot in order to produce subplots, or just one. Axes will automatically inherit their limits from the data plotted, or can be manually set.
fig = plt.figure(figsize=(9.5, 8))
ax = plt.axes()
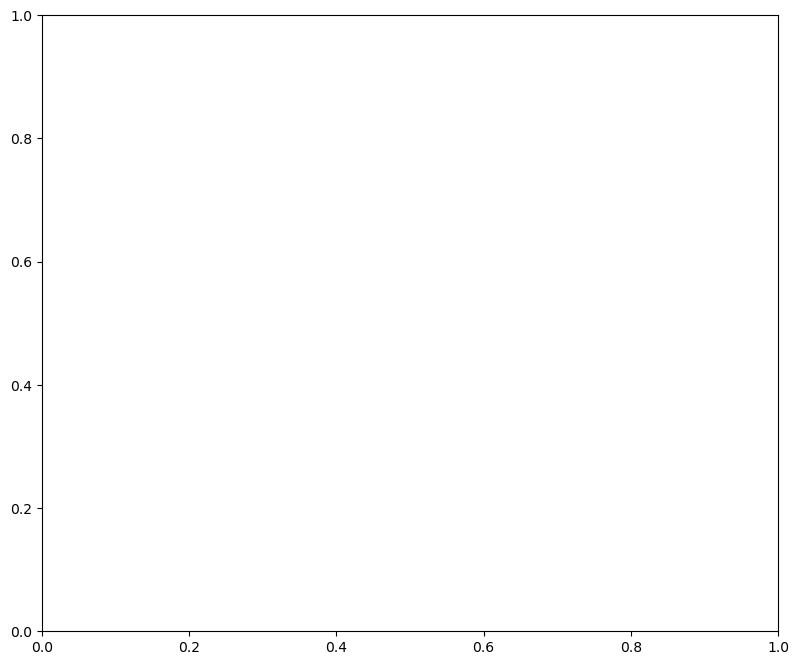
Plot
Adding the data to the figure can be done through several different plot types: line, contour, bar, histogram. Here we use two line plots:
fig = plt.figure(figsize=(9.5, 8))
ax = plt.axes()
ax.plot(x,y1)
ax.plot(x,y2);
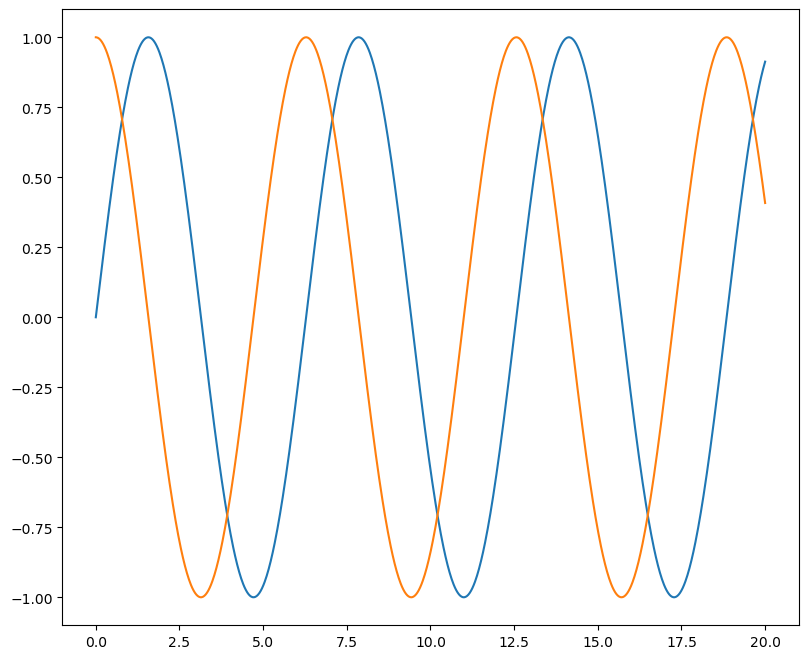
Titles and Labels
Titles and labels are important for indicating what the figure is plotting. It is a good idea to include relevant units in your axis labels.
fig = plt.figure(figsize=(9.5, 8))
ax = plt.axes()
ax.plot(x,y1)
ax.plot(x,y2)
ax.set_title("Dummy Data")
ax.set_xlabel("X (radians)");
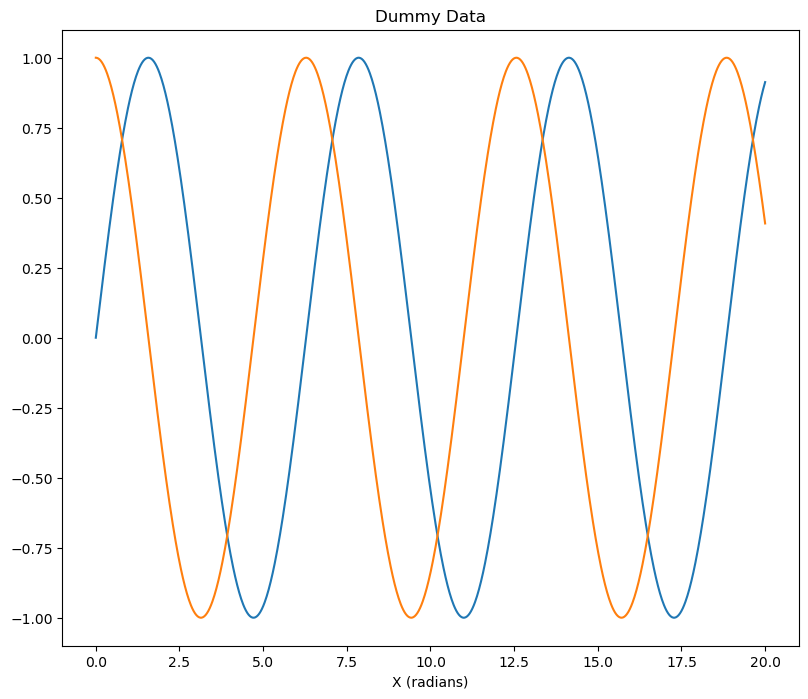
Legends
If you’re plotting multiple lines of data, it’s a good idea to include a legend. Here is how you call or point to the legend:
fig = plt.figure(figsize=(9.5, 8))
ax = plt.axes()
ax.plot(x,y1,label='sine')
ax.plot(x,y2,label='cosine')
ax.set_title("Dummy Data")
ax.set_xlabel("X (radians)")
plt.legend(loc="upper left");
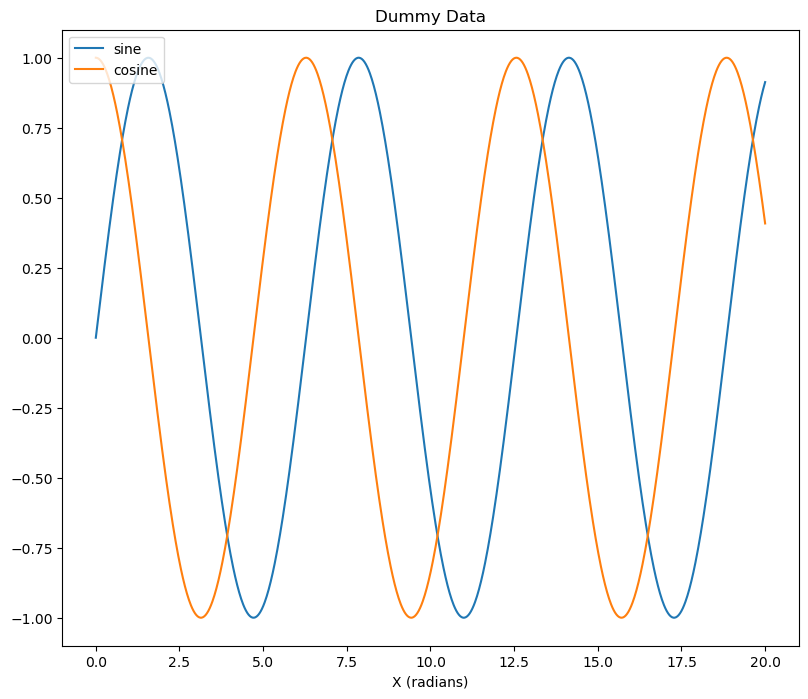
Colorbars
While legends are more appropriate for line or bar plots, colorbars are most commonly used for contour plots and sometimes to apply a third level of dimension to a scatter plot.
Let’s shift our example to better demonstrate a colorbar by workign with a filled contour plot:
# Generate dummy data
data = [[1, 4, 5, 6, 8.2],
[9, 8.4, 10, 10.6, 9.7],
[4.4, 5, 0, 6.6, 1.4],
[4.6, 5.2, 1.5, 7.6, 2.4]]
# Convert data into type xarray.DataArray
data = xr.DataArray(data,
dims=["lat", "lon"],
coords=dict(lat=np.arange(4), lon=np.arange(5)))
data
<xarray.DataArray (lat: 4, lon: 5)> Size: 160B array([[ 1. , 4. , 5. , 6. , 8.2], [ 9. , 8.4, 10. , 10.6, 9.7], [ 4.4, 5. , 0. , 6.6, 1.4], [ 4.6, 5.2, 1.5, 7.6, 2.4]]) Coordinates: * lat (lat) int64 32B 0 1 2 3 * lon (lon) int64 40B 0 1 2 3 4
fig = plt.figure(figsize=(9.5, 8))
ax = plt.axes()
pcm = ax.contourf(data,cmap='viridis')
ax.set_title("Dummy Data")
ax.set_xlabel("Longitude (\N{DEGREE SIGN})")
ax.set_ylabel("Latitude (\N{DEGREE SIGN})")
fig.colorbar(pcm,ax=ax);
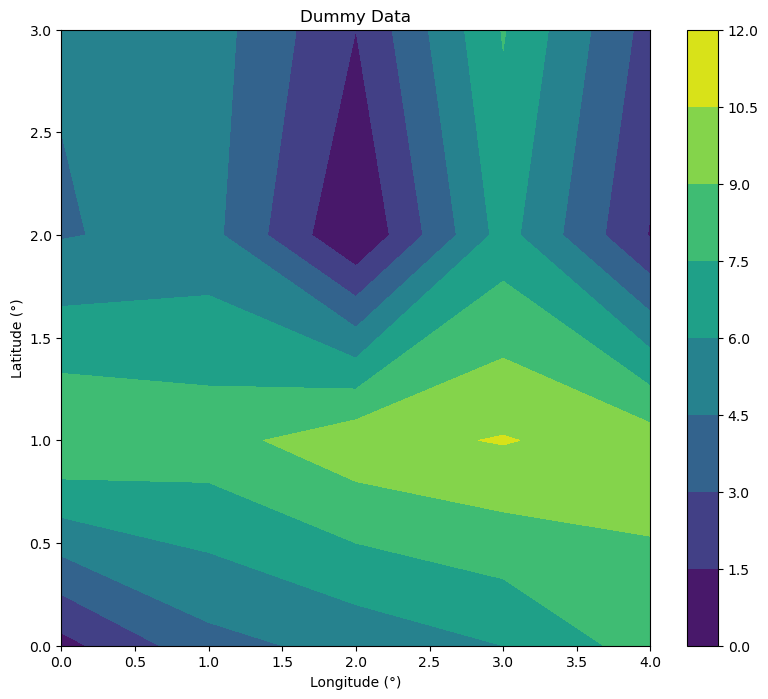
Annotations
Additional annotations allow you to specify some text and a location to indicate almost anything.
Here we use GeoCAT-viz to add annotations to the maxima in a contour plot:
fig = plt.figure(figsize=(9.5, 8))
ax = plt.axes()
pcm = ax.contourf(data,cmap='viridis')
ax.set_title("Dummy Data")
ax.set_xlabel("Longitude (\N{DEGREE SIGN})")
ax.set_ylabel("Latitude (\N{DEGREE SIGN})")
fig.colorbar(pcm,ax=ax)
# Find local maximum with GeoCAT-Viz find_local_extrema
lmax = gv.find_local_extrema(data, eType='High')[0]
# Plot labels for local mins
max_value = data.data[lmax[1]][lmax[0]]
ax.text(lmax[0], lmax[1],'Maxima = '+str(max_value))
# Show plot
plt.show();
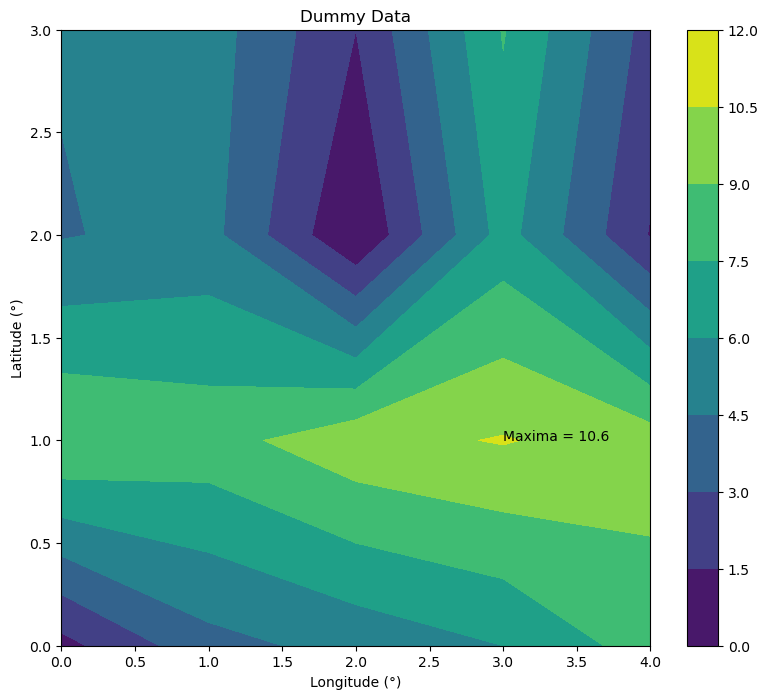
Summary
There are several key elements to a Python plot and knowing what they are called is instrumental to begin your journey for further customization.
What’s next?
Next up, the specialty plots called Taylor Diagrams.