Use xrefcoord
to Generate Coordinates
When using Kerchunk
to generate reference datasets for GeoTIFF’s, only the dimensions are preserved. xrefcoord
is a small utility that allows us to generate coordinates for these reference datasets using the geospatial metadata. Similar to other accessor add-on libraries for Xarray
such as rioxarray
and xwrf
, xrefcord
provides an accessor for an Xarray
dataset. Importing xrefcoord
allows us to use the .xref
accessor to access additional methods.
In this tutorial we will use the generate_coords
method to build coordinates for the Xarray
dataset. xrefcoord
is very experimental and makes assumptions about the underlying data, such as each variable shares the same dimensions etc. Use with caution!
Overview
Within this notebook, we will cover:
How to load a Kerchunk reference dataset created from a collection of GeoTIFFs
How to use
xrefcoord
to generate coordinates from a GeoTIFF reference dataset
Prerequisites
Concepts |
Importance |
Notes |
---|---|---|
Required |
Core |
|
Required |
Core |
Time to learn: 45 minutes
import xarray as xr
import xrefcoord # noqa
storage_options = {
"remote_protocol": "s3",
"skip_instance_cache": True,
} # options passed to fsspec
open_dataset_options = {"chunks": {}} # opens passed to xarray
ds = xr.open_dataset(
"references/RADAR.json",
engine="kerchunk",
storage_options=storage_options,
open_dataset_options=open_dataset_options,
)
/home/runner/miniconda3/envs/kerchunk-cookbook/lib/python3.10/site-packages/kerchunk/xarray_backend.py:46: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
return xr.open_dataset(m, engine="zarr", consolidated=False, **open_dataset_options)
/home/runner/miniconda3/envs/kerchunk-cookbook/lib/python3.10/site-packages/kerchunk/xarray_backend.py:46: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
return xr.open_dataset(m, engine="zarr", consolidated=False, **open_dataset_options)
# Generate coordinates from reference dataset
ref_ds = ds.xref.generate_coords(time_dim_name="time", x_dim_name="X", y_dim_name="Y")
# Rename to rain accumulation in 24 hour period
ref_ds = ref_ds.rename({"0": "rr24h"})
/home/runner/miniconda3/envs/kerchunk-cookbook/lib/python3.10/site-packages/xrefcoord/coords.py:5: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ds[x_dim_name] = coord_dict["x"]
/home/runner/miniconda3/envs/kerchunk-cookbook/lib/python3.10/site-packages/xrefcoord/coords.py:6: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ds[y_dim_name] = coord_dict["y"]
/home/runner/miniconda3/envs/kerchunk-cookbook/lib/python3.10/site-packages/xrefcoord/coords.py:7: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
return ds.rename({x_dim_name: "x", y_dim_name: "y"})
/tmp/ipykernel_4152/4188701970.py:4: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ref_ds = ref_ds.rename({"0": "rr24h"})
Create a Map
Here we are using Xarray
to select a single time slice and create a map of 24 hour accumulated rainfall.
ref_ds["rr24h"].where(ref_ds.rr24h < 60000).isel(time=0).plot(robust=True)
/tmp/ipykernel_4152/2314829678.py:1: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ref_ds["rr24h"].where(ref_ds.rr24h < 60000).isel(time=0).plot(robust=True)
/tmp/ipykernel_4152/2314829678.py:1: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ref_ds["rr24h"].where(ref_ds.rr24h < 60000).isel(time=0).plot(robust=True)
<matplotlib.collections.QuadMesh at 0x7fe6cbdb7be0>
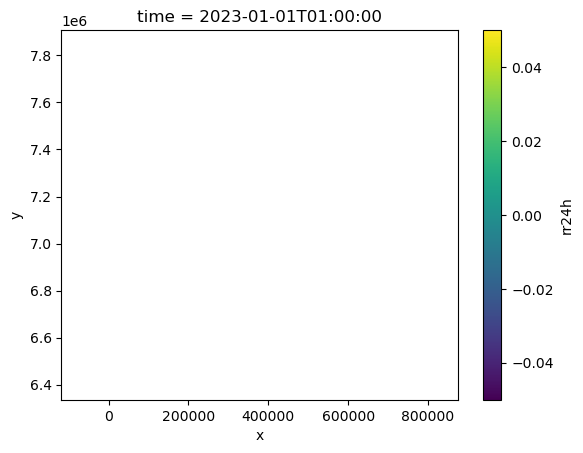
Create a Time-Series
Next we are plotting accumulated rain as a function of time for a specific point.
ref_ds["rr24h"][:, 700, 700].plot()
/tmp/ipykernel_4152/4079125503.py:1: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ref_ds["rr24h"][:, 700, 700].plot()
/tmp/ipykernel_4152/4079125503.py:1: UserWarning: Converting non-nanosecond precision datetime values to nanosecond precision. This behavior can eventually be relaxed in xarray, as it is an artifact from pandas which is now beginning to support non-nanosecond precision values. This warning is caused by passing non-nanosecond np.datetime64 or np.timedelta64 values to the DataArray or Variable constructor; it can be silenced by converting the values to nanosecond precision ahead of time.
ref_ds["rr24h"][:, 700, 700].plot()
[<matplotlib.lines.Line2D at 0x7fe6c9536860>]
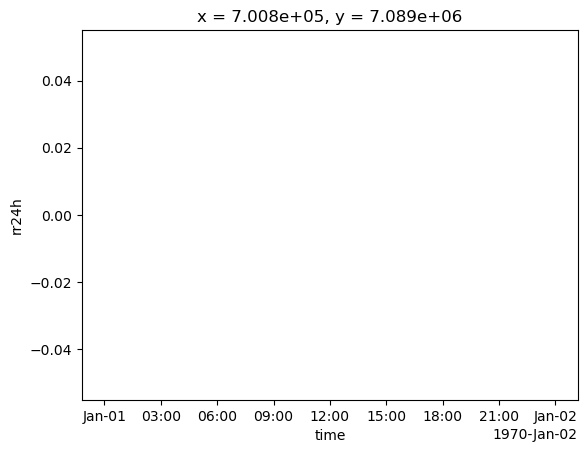